.NET MAUI Dialog Service
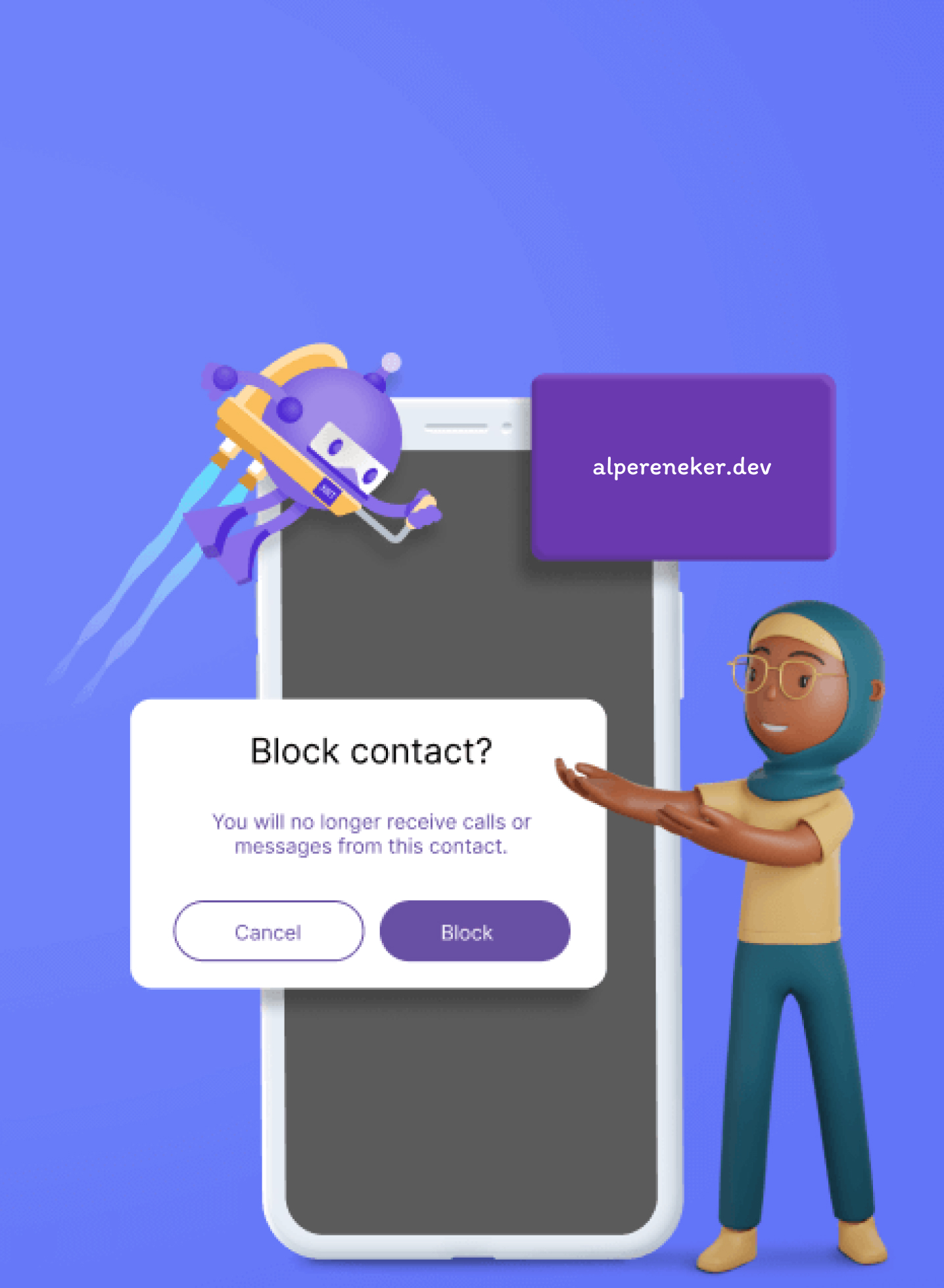
Simplifying User Interaction with .NET MAUI DialogService
In mobile app development, user interaction is crucial for a smooth and engaging experience. .NET MAUI, a cross-platform framework for building modern mobile apps, provides built-in functionalities to streamline this interaction. One such feature is the DialogService
.
This blog post explores the DialogService
in .NET MAUI, explaining its role and how it can simplify displaying various types of dialogs within your app.
What is DialogService?
The DialogService
is an abstraction layer built on top of the native platform dialogs. It provides a consistent and centralized way to display different types of message boxes to users, regardless of the underlying platform (Android, iOS, etc.).
By leveraging DialogService
, you can:
- Display simple alerts with titles and messages.
- Prompt users for confirmation with custom button captions.
- Request user choices with accept and cancel options.
Let’s look at an example:
Implementing DialogService
While .NET MAUI offers built-in methods like DisplayAlert
and DisplayActionSheet
for showing popups, DialogService
provides a more decoupled and testable approach. Here’s an example implementation of a basic DialogService
:
DialogService.cs
public class DialogService : IDialogService
{
public Task PromptAsync(string title, string message, string? confirm = null)
{
confirm ??= AppStrings.Confirm; // Set default confirmation text
return Application.Current!.MainPage!.DisplayAlert(title, message, confirm);
}
public Task<bool> RequestAsync(string title, string message, string? accept = null, string? cancel = null)
{
accept ??= AppStrings.Accept; // Set default accept text
cancel ??= AppStrings.Cancel; // Set default cancel text
return Application.Current!.MainPage!.DisplayAlert(title, message, accept, cancel);
}
}
MauiProgram.cs
builder.Services
.AddSingleton<IDialogService, DialogService>()
return builder;
xxViewModel.cs
public LoginViewModel(IDialogService dialogService)
{
_dialogService = dialogService;
}
private async Task LoginTask()
{
var result = await _userService.Login(userName, password);
if (result == null)
{
_= _dialogService.PromptAsync("Uyarı!", "Kullanıcı adı veya şifre yanlış!");
return;
}
}
This implementation showcases two methods:
PromptAsync
: Displays a confirmation dialog with a title, message, and a single confirmation button. You can optionally provide a custom confirmation button text.RequestAsync
: Displays a choice dialog with a title, message, and two buttons: accept and cancel. You can optionally customize the text for both buttons.
Benefits of Using DialogService
- Centralized Control: Manages all dialogs from one place, making your code easier to maintain and test.
- Platform Independence: Provides a consistent approach to displaying dialogs across different platforms.
- Flexibility: Allows customization of button captions for a tailored user experience.
- Testability: Easier to write unit tests for dialog interactions compared to direct usage of platform-specific methods.
Conclusion
The DialogService
in .NET MAUI offers a convenient way to manage user interactions through dialogs. It promotes code reusability, platform independence, and facilitates better testability. By incorporating this service into your .NET MAUI app development, you can create a more user-friendly and interactive experience for your mobile users.
Further Exploration:
- Explore the possibility of extending
DialogService
to support additional dialog types like progress bars or custom content dialogs. - Consider implementing dependency injection for a more loosely coupled and testable approach.
Feel free to customize this draft with your own insights and examples. You can also add information about potential limitations or advanced usage scenarios of DialogService
.
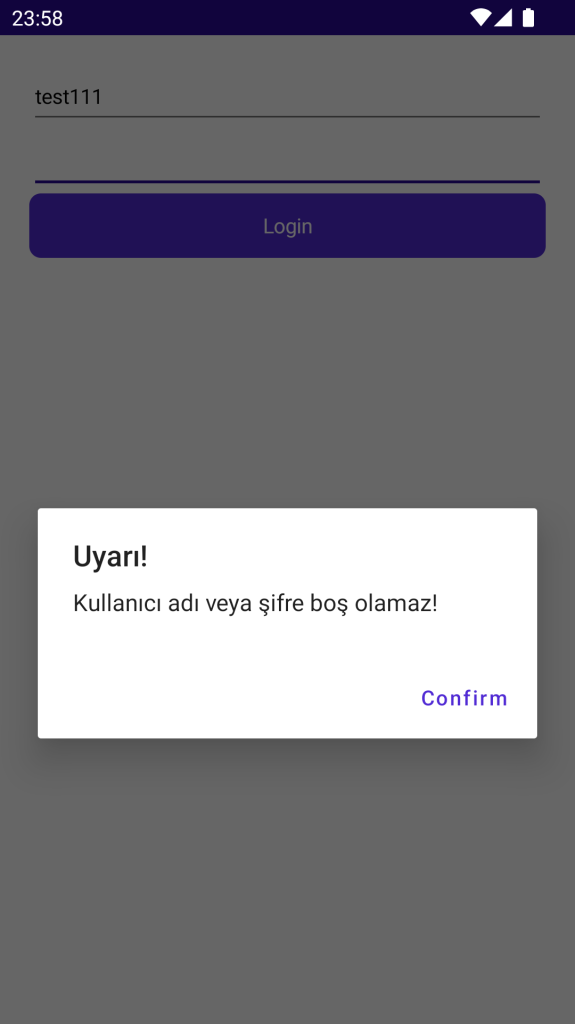
Thanks;
https://learn.microsoft.com/en-us/dotnet/maui/user-interface/pop-ups?view=net-maui-8.0
and Gemini 🙂